Azure Infrastructure as Code - Part Six
Are you ready to wrap this up? In Azure Infrastructure as Code - Part Six we are going to put everything together and generate a report that can be presented to small to medium sized businesses on their cloud security posture. First, we are going to be analyzing Terraform code with Checkov. So let's do that.
Make Terraform Directory and Move There
mkdir ~/wrappingup
cd ~/wrappingup
Create main.tf file with VS Code
code main.tf
Paste Code into File, and Save
terraform {
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "3.90.0"
}
}
}
provider "azurerm" {
# Configuration options
features {
}
}
variable "prefix" {
default = "tpot"
}
resource "azurerm_resource_group" "tpot-rg" {
name = "${var.prefix}-resources"
location = "East US"
}
resource "azurerm_virtual_network" "main" {
name = "${var.prefix}-network"
address_space = ["10.0.0.0/16"]
location = azurerm_resource_group.tpot-rg.location
resource_group_name = azurerm_resource_group.tpot-rg.name
}
resource "azurerm_subnet" "internal" {
name = "internal"
resource_group_name = azurerm_resource_group.tpot-rg.name
virtual_network_name = azurerm_virtual_network.main.name
address_prefixes = ["10.0.2.0/24"]
}
resource "azurerm_virtual_machine" "main" {
depends_on = [ azurerm_resource_group.tpot-rg ]
name = "${var.prefix}-vm"
location = azurerm_resource_group.tpot-rg.location
resource_group_name = azurerm_resource_group.tpot-rg.name
network_interface_ids = [azurerm_network_interface.tpot-vm-nic.id]
vm_size = "Standard_A2m_v2"
# Uncomment this line to delete the OS disk automatically when deleting the VM
delete_os_disk_on_termination = true
# Uncomment this line to delete the data disks automatically when deleting the VM
delete_data_disks_on_termination = true
storage_image_reference {
publisher = "canonical"
offer = "ubuntu-24_04-lts"
sku = "minimal-gen1"
version = "latest"
}
storage_os_disk {
name = "tpot-disk"
caching = "ReadWrite"
create_option = "FromImage"
managed_disk_type = "Standard_LRS"
}
os_profile {
computer_name = "hostname"
admin_username = "azureuser"
admin_password = "CyberNOW!"
}
os_profile_linux_config {
disable_password_authentication = false
}
}
# Create Security Group to access linux
resource "azurerm_network_security_group" "tpot-nsg" {
depends_on=[azurerm_resource_group.tpot-rg]
name = "linux-vm-nsg"
location = azurerm_resource_group.tpot-rg.location
resource_group_name = azurerm_resource_group.tpot-rg.name
security_rule {
name = "AllowALL"
description = "AllowALL"
priority = 100
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "*"
source_address_prefix = "Internet"
destination_address_prefix = "*"
}
security_rule {
name = "AllowSSH"
description = "Allow SSH"
priority = 150
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "22"
source_address_prefix = "Internet"
destination_address_prefix = "*"
}
}
# Associate the linux NSG with the subnet
resource "azurerm_subnet_network_security_group_association" "tpot-vm-nsg-association" {
depends_on=[azurerm_resource_group.tpot-rg]
subnet_id = azurerm_subnet.internal.id
network_security_group_id = azurerm_network_security_group.tpot-nsg.id
}
# Get a Static Public IP
resource "azurerm_public_ip" "tpot-vm-ip" {
depends_on=[azurerm_resource_group.tpot-rg]
name = "tpot-vm-ip"
location = azurerm_resource_group.tpot-rg.location
resource_group_name = azurerm_resource_group.tpot-rg.name
allocation_method = "Static"
}
# Create Network Card for linux VM
resource "azurerm_network_interface" "tpot-vm-nic" {
depends_on=[azurerm_resource_group.tpot-rg]
name = "tpot-vm-nic"
location = azurerm_resource_group.tpot-rg.location
resource_group_name = azurerm_resource_group.tpot-rg.name
ip_configuration {
name = "internal"
subnet_id = azurerm_subnet.internal.id
private_ip_address_allocation = "Dynamic"
public_ip_address_id = azurerm_public_ip.tpot-vm-ip.id
}
}
output "public_ip" {
value = azurerm_public_ip.tpot-vm-ip.ip_address
}
Format the file
terraform fmt
Execute Checkov
Make sure you're in the directory that your Terraform is in.
checkov -f main.tf
Results
We have seven failed checks. Looking through the list it is warning us for stuff that we have configured specifically like ports that are exposed to the public internet. Since this is the honeypot that we just configured in Azure Cybersecurity Labs - Part Four, we know that this works and we know that this is how it needs to be configured to work properly.
So let's go ahead and deploy this to Azure.
Type az login in the terminal to establish your credentials if they aren't cached already.
az login
Initialize the directory
terraform init
Now terraform plan
terraform plan
Note: Take a look at the Terraform Plan and see the 8 resources that we are creating. While not mandatory, it's good practice to 'Terraform Plan' to review your changes BEFORE deploying.
Now terraform apply
terraform apply
Make sure you have previously deleted this project from Azure so that you can deploy it again.
Prowler
Now we're getting into to new stuff. Prowler is an Open Source security tool to perform AWS, Azure, Google Cloud and Kubernetes security best practices assessments, audits, incident response, continuous monitoring, hardening and forensics readiness, and also remediations! We have Prowler CLI (Command Line Interface) that we call Prowler Open Source.
You can install Prowler using Pip3 like we did with Checkov in Azure Cybersecurity Labs - Part Five. So let's do that.
pip3 install prowler
and then we run Prowler
prowler azure --az-cli-auth
The results are displayed on your screen and also exported to your 'output directory'
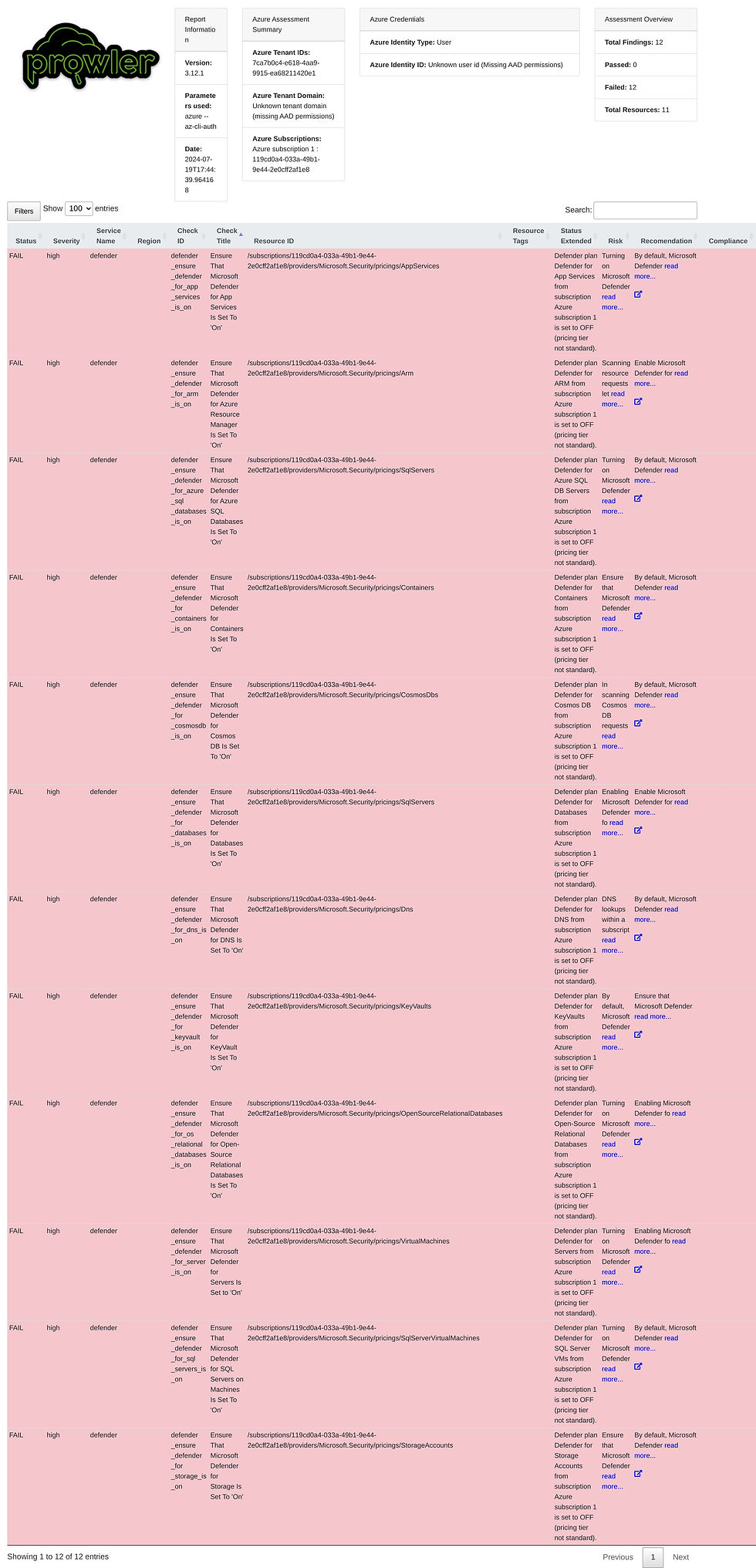
I like view the HTML file and the use a html to jpg or html to pdf converter online. Our environment is a new environment so it doesn't have much on here other than turning Microsoft Defender on for our resources which we do not currently have deployed. Using Prowler is very simple and the value that you are adding as a freelancer is discerning the results and narrowing it down for the business to what is useful and actionable to them.
Do not just give them this report and be done with it. They will be unhappy. Instead write specific recommendations in your own report with your own template with step-by-step instructions on how to fix each issue that is important to them.
And that wraps up the Azure Cybersecurity Labs series but stick around for one BONUS as we discuss Serverless computing.
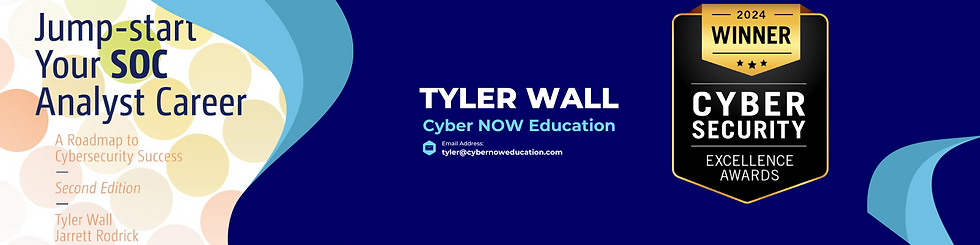
Tyler Wall is the founder of Cyber NOW Education. He holds bills for a Master of Science from Purdue University and also CISSP, CCSK, CFSR, CEH, Sec+, Net+, and A+ certifications. He mastered the SOC after having held every position from analyst to architect and is the author of three books, 100+ professional articles, four online courses, and regularly holds webinars for new cybersecurity talent.
You can connect with him on LinkedIn.
To view my dozens of courses, visit my homepage and watch the trailers!
Become a Black Badge member of Cyber NOW® and enjoy all-access for life.
Check out my latest book, Jump-start Your SOC Analyst Career: A Roadmap to Cybersecurity Success, winner of the 2024 Cybersecurity Excellence Awards.
Comments